When and How to Use PowerShell Not Equal
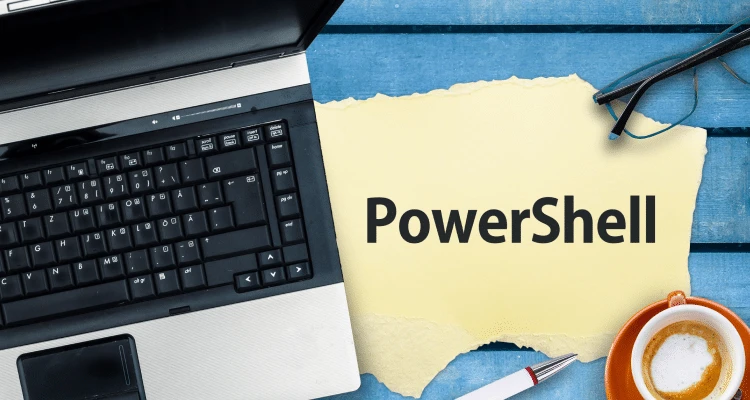
Powershell Not Equal Command is a powerful tool that can compare values in various ways. The syntax for the Not Equal operator is “-ne”. It can compare strings, integers, and other data types. By using the Not Equal operator, you can quickly compare two values to see if they are not equal to each other.
This guide will provide an overview of the Powershell Not Equal Command, including how to use it in a command line, examples of how to use it for specific tasks, and how to use it in various scenarios.
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell for Microsoft Windows. It provides a command-line environment for automating tasks and managing systems. PowerShell enables administrators to perform administrative tasks on both local and remote Windows systems.
It is based on the .NET framework, and it includes an extensive set of cmdlets, providers, functions, scripts, and a powerful scripting language to provide an integrated scripting experience. PowerShell is designed to be used by system administrators and IT professionals to automate and simplify their everyday tasks.
It provides a comprehensive set of tools and features for managing and configuring Windows systems, including managing the Windows registry, managing Windows services, creating scheduled tasks, and performing complex web and network administration tasks. PowerShell provides different comparison operators to compare values, objects, variables, outputs, etc.
PowerShell operators are divided into 8 groups. These are arithmetic operators, assignment operators, comparison operators, logical operators, redirectional operators, spilt and join operators, type operators, and unary operators.
Prerequisites:
To understand this tutorial better, you will need to install PowerShell 7 and meet the Windows 10 system requirements.
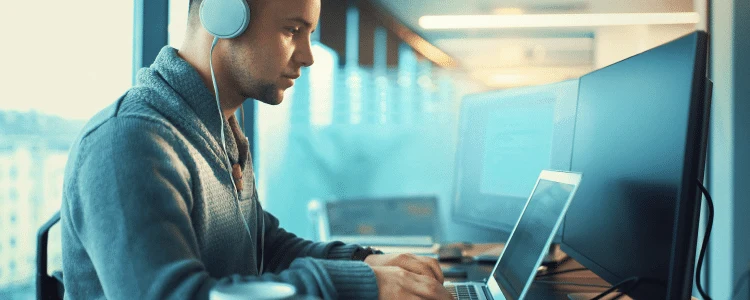
Basics of Using PowerShell Not Equal Operator
The -ne operator in PowerShell evaluates if the value of two objects is not equal and provides a Boolean value, with True indicating the values are not the same and False indicating they are the same. However, let’s go through how and when to use the PowerShell Not Equal operator.
Comparing Variables
Comparison of two variables is one of the most popular uses of the PowerShell Not Equal operator. For instance, let’s say you want to check to see if two variables, $a and $b, are equal; you will use the comparison syntax below:
$a -ne $b
This comparison will return Results if $a and $b do not have equal values. If not, it will return False.
Follow the steps below to compare values using PowerShell Not Equal operator
Step 1: Before we begin, you will need to construct a variable that will be used as an example in this tutorial. To do this, use your PowerShell console to execute the command below.
The variables $a, $b, $c, and $d each represent a different value. To determine whether these values are equal, you have to compare each one of them.
To demonstrate how the PowerShell Not Equal operator functions with equal values, you set $c and $d to the same value.
$a = 1
$b = 2
$c = 3
$d = 3
Step 2: To determine whether the values of $a and $b are equal or not, use the PowerShell Not Equal comparison.
$a -ne $b
Since $a has a value of 1 and $b of 2, which are not equal, the result is True.
Given that the values of $c and $d are equal (3=3), you will observe a False output return, as displayed in the image above. Variables with unequal values
Step 3: Also, compare variables $c and $d to determine if they are not equal.
$c -ne $d
Given that the values of $c and $d are equal (3=3), you will observe a False output return, as displayed in the image below
Comparing Values
You can compare multiple values directly with the PowerShell Not Equal operator when objects are not in variables.
For instance, the command below uses the PowerShell Not Equal operator to compare two values explicitly.
5 -ne 3
Because the values aren’t equal, a True value will be returned, as illustrated below.
Contrarily, comparing equal values will result in a False being returned.
5 -ne 5
Removing a Value from the Array
When two values are not equal, the PowerShell Not Equal operator gives a Boolean result. In addition to that feature, this operator can exclude certain elements from an array.
For instance, construct a variable called $numbers that holds an array of the digits 1 through 5.
# Create an array containing numbers 1 through 5.
$numbers = 1..5
# Display the array
$numbers
Run the following command after that to return the array with the exception of one number (4)
$numbers -ne 4
You can see that, with the exception of the number 4, the command returned all of the numbers in the array.
Comparing String Variables
Asides from using the PowerShell Not Equal operator to compare numbers, you can also use it to compare strings. Follow the steps below:
Step 1: Run the command below to see if two strings, “Hello” and “World,” are not equal.
"Hello" -ne "World"
Since “Hello” and “World” are not equal, the result is True.
Step 2: Compare to determine if the strings “Hello” and “hello” are equal.
"Hello" -ne "hello"
As you can see, the outcome is False since “Hello” is equal to “hello”.
Step 3: There is no case sensitivity with the PowerShell Not Equal operator. The preceding step showed that “hello” is equal to “hello.” But instead of using the -ne operator, use the -cne operator, which stands for Case Sensitive Not Equal, to compare string values if case sensitivity is necessary.
"Hello" -cne "hello"
Therefore, PowerShell no longer considers “Hello” and “hello” to be equal.
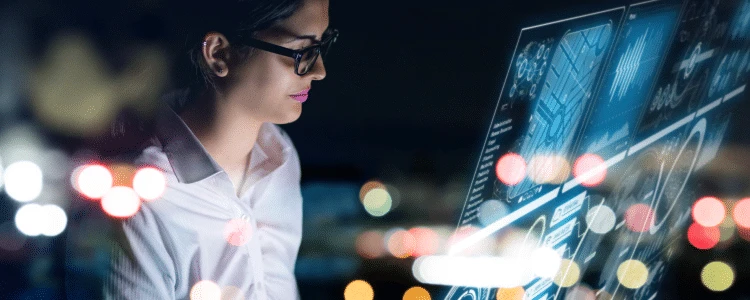
PowerShell Not Equal Operator in the Real World with examples
This section puts PowerShell not equal operator into use in the real world. However, let’s consider the following below:
Monitoring Services Status
Monitoring server service status is a fundamental aspect of Windows systems administration. In order to complete this process, the services whose Status Is Not Running must be filtered out.
For instance, use the Get-Service cmdlet to see a list of every service installed on your machine.
Get-Service
The output listed below shows both operating and halted services.
Run the command below and use the -ne operator to filter the services that are not running.
Get-Service | Where-Object {$_.Status -ne "Running"}
After using the PowerShell Not Equal comparison, as seen below, the Status column only displays the services that have been halted.
In the same way, use the command below to retrieve the services whose Status is Not Stopped.
Get-Service | Where-Object {$_.Status -ne "Stopped"}
However, only services that are currently active are visible.
Combining PowerShell Not Equal with the AND Operator
The PowerShell -and operator allow you to pair the Not Equal condition with another comparison operator, such as -eq (equal). This approach is helpful if the object you’re comparing needs to satisfy several requirements.
A standard requirement for monitoring server services, for instance, is that all Automatic services be active. Run the command below to get a list of all services with the Status Not Running and StartType Automatic flags. In this case, the object value has to satisfy every requirement.
Get-Service |
Where-Object {$_.Status -ne "Running" -and $_.StartType -eq "Automatic"} |
Select-Object Status,StartType,Name
You can now identify the automatic services that are not active, which can guide your decision-making.
If/Else Conditional Logic
You can use the PowerShell Not Equal operator in the if/else statements and other conditional expressions. A service, for instance, must normally be active if its StartType value is Automatic.
The following script receives a list of all services, determines which automatic services are not running, and then performs the necessary restorative steps to start the service and display a notice.
Start-Service $_. Name is commented out and won’t execute. To run the command, remove the comment from this line.
Use PowerShell to execute the code below.
Get-Service -ErrorAction SilentlyContinue | ForEach-Object {
if ($_.Status -ne 'Running' -and $_.StartType -eq 'Automatic') {
"The service [$($_.Name)] StartType is [$($_.StartType)] but its current Status is [$($_.Status)]"
# Start-Service $_.Name
}
}
Your result should display any automatic services that are not active.
Conclusion
PowerShell Not Equal Command is an incredibly powerful and versatile tool that can be used to automate almost any task, manage systems and networks, and produce reports. With its wide range of features, PowerShell can help you efficiently manage and automate tasks. Learning how to use PowerShell is a worthwhile investment of time and effort, but with a bit of practice, you can easily become a PowerShell expert and use PowerShell to its fullest potential.
ServerMania offers dozens of operating systems to ensure you have the right software to learn PowerShell. We offer Windows Dedicated Server and various free Linux options for your device. Contact us today to get more information about our products and services.
Was this page helpful?